Game Programming and Logic for the Robber/Cactus
- Katherine Neul
- Apr 7, 2021
- 5 min read
Updated: Apr 9, 2021
This blog post is going to revolve more around the coding applying to the Robber over the Cactuses, the Cactuses are just static objects that can kill the Cowboy. The Robber is going to be a prolling Enemy which basically means they will be walking left to right on a stretch of ground that can kill the Cowboy by walking into him.
Patrolling:-
The variables I need to make the patrolling script work are a movement speed for the rate at which the Robber walks, I need to reference the rigid body to allow the sprite to move under the use of physics. I need the ground in which the Robber will be patrolling and so that the code knows where the Robber is meant to be patrolling and I need a raycast hit that finds where the ground ends.
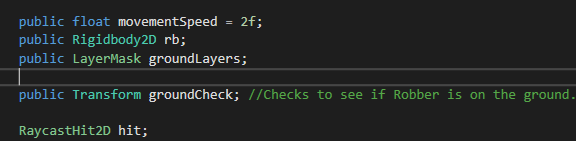
This script basically takes the groundCheck game object and adds an invisible line to it, the line scans the area in front which will be a layer that is ground and when the Robber moves along the ground to the point where the ground stops the Robber will change the direction in which they are moving towards to the opposite, direction and do the same thing until they reach the other side of the ground then it will go the other direction again.

As the Robber is walking on Entry to the scene and will always be walking unless he is hurt or dead; I don't actually need to tell Unity to make the Robber walk but I do need to tell Unity to flip the animation according to what direction the Robber is walking towards.
If the 'raycast hit' is not equal to false; so if the raycast detects the ground or is hitting the ground the script tells the Robber to move left or right along the ground but if the Robber is not on the ground he will not move left or right. Then if the Robber is on the ground as is facing towards the right direction then he will move towards the right and if the Robber is not looking towards the right then he will move towards the left. Then if there is another scenario such as the Robber running out of ground space and changing direction then the animation will flip making the Robber not look right and then the Robber will move not towards the right which is towards the left- this makes him look left when moving left and look right when moving right. The Robber is defaulted to always looking towards the right.
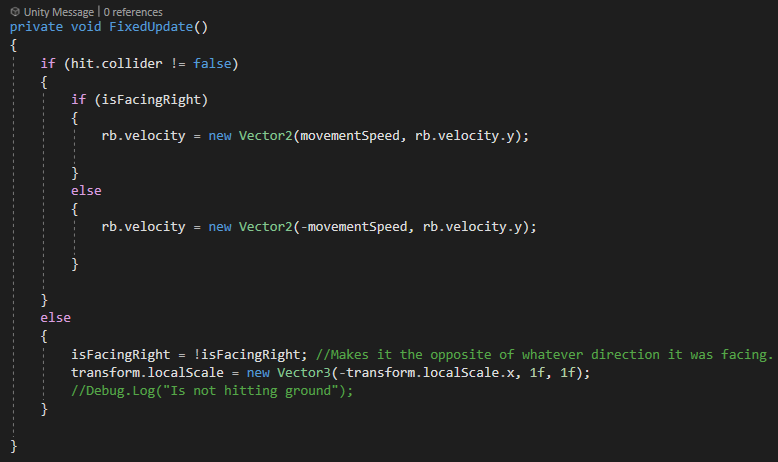
This is placed in the FixedUpdate() because FixedUpdate() can be called multiple times per frame meaning that the situation regarding the code can be ever-changing in comparison to it being placed in the Update() where the functions would only be called once per frame meaning that it could only be one situation or another.
Taking Damage:-
These are the variables that I need so that the Robber can take damage/ be attacked. The process that is linked to to the Robber taking damage is the Attack() function that was written in the script for Player/Cowboy Movement. In the Player Movent script, the damage dealt to the Robber by the player is 20 which is constant, meaning that every time the Cowboy hits/attacks the Robber the health of the Robber will decrease by 20; with a bit of simple math this equivalates to around 5 hits to get the Robber to 0. The variable currentHealth is a value that updates every time the Robber is hit.
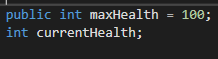
As the game starts the Robber's health is instantly set to 100 but the Robber's health will only be 100 until he is attacked by the Cowboy. Then when the Robber is attacked by the Cowboy he will take damage at a rate of minus 20 (this deducts from currentHealth), for each time that the player Takes Damage the animation for the Robber being hurt will take place, and on the 5th hit the Robber should have currentHealth equal to 0.

If and when currentHealth is equal to 0 or less the Robber will Die/ the script will run the function Die().
Death:-
The function Die() will only run if the currentHealth of the Robber is equal 0 is less as shown is the Take Damage() function. When the Robber dies the debug will tell the player and the Animator will play the Robber Dead animation, the Robber will then disappear and the script linked to the Robber that has died will stop working/ will not be activated.

Do Not Collide With Cacti:-
I suppose I made a minor mistake so it's not really a bug but, one of the platforms that the enemies patrol on has a cactus on it. The issue with that is the cactus has a box collider on it so that if the player collides with it the Cowboy will die but one of the Robber was also colliding with it too. This bit of code tells the Enemies to ignore each other's colliders but only Enemy colliders are to be ignored. This makes it so that the Enemy can still patrol on the ground without bumping into the cactuses or even each other. This script would be very useful for future scenes where I could have two Robbers on one piece of ground and they wouldn't collide.
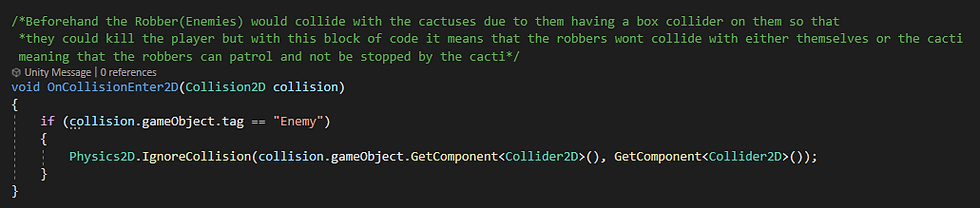
Bugs:-
Genuinely one of the only bugs I had with this game and it became a bug because of what I wanted the code to do. I wanted it so that when the Enemy dies the Enemy would play the animation for the Robber being dead and the Robber would just lie dead in the scene. I made it so that if the Robber just lay dead in the scene the Cowboy wouldn't collide with the Robber by disabling the Box Collider attached to the Robber, it's just that this makes the Robber fall through the floor because the Box Collider isn't there. After discovering that the Robber falls through the floor when he dies I decided to just settle on destroying the Robber completely and disabling it's script which works a lot better.

This screenshot is to demonstrate how I linked the variables to what they needed to be linked to, this made the scripts work how they were intended to work.
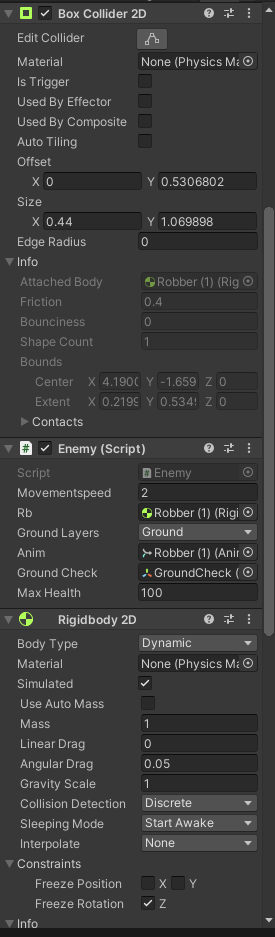
Enemy Control
public class Enemy : MonoBehaviour
{
public float speed = 2f;
public Rigidbody2D rb;
public LayerMask groundLayers;
public Animator anim; //Reference to the animator
public Transform groundCheck; //Checks to see if Robber is on the ground.
bool isFacingRight = true;
RaycastHit2D hit;
public int maxHealth = 100;
int currentHealth;
void Start()
{
currentHealth = maxHealth;
}
public void TakeDamage(int damage)
{
currentHealth -= damage;
anim.SetTrigger("Hurt");
if(currentHealth <= 0)
{
Die();
}
}
private void Update()
{ //Enemy will patrol the ground space they are given when they are on the ground.
hit = Physics2D.Raycast(groundCheck.position, -transform.up, 1f, groundLayers);
}
private void FixedUpdate()
{
if (hit.collider != false)
{
if (isFacingRight)
{
rb.velocity = new Vector2(speed, rb.velocity.y);
}
else
{
rb.velocity = new Vector2(-speed, rb.velocity.y);
}
}
else
{
isFacingRight = !isFacingRight; //Makes it the opposite of whatever direction it was facing.
transform.localScale = new Vector3(-transform.localScale.x, 1f, 1f);
//Debug.Log("Is not hitting ground");
}
}
void Die()
{
Debug.Log("Enemy Died!");
anim.SetBool("isDead", true);
Destroy(gameObject);
//GetComponent<BoxCollider2D>().enabled = false;
this.enabled = false;
}
/*Beforehand the Robber(Enemies) would collide with the cactuses due to them having a box collider on them so that
*they could kill the player but with this block of code it means that the robbers wont collide with either themselves or the cacti
meaning that the robbers can patrol and not be stopped by the cacti*/
void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.tag == "Enemy")
{
Physics2D.IgnoreCollision(collision.gameObject.GetComponent<Collider2D>(), GetComponent<Collider2D>());
}
}
}
Comments